C++ Language
- Home
- Courses
- C++ Language
Introduction to C++ Language
The C++ language course covers basics to advanced topics, including object-oriented programming, data structures, STL, file handling, multithreading, and optimization, providing a solid foundation for robust application development.
Duration - 4 Months
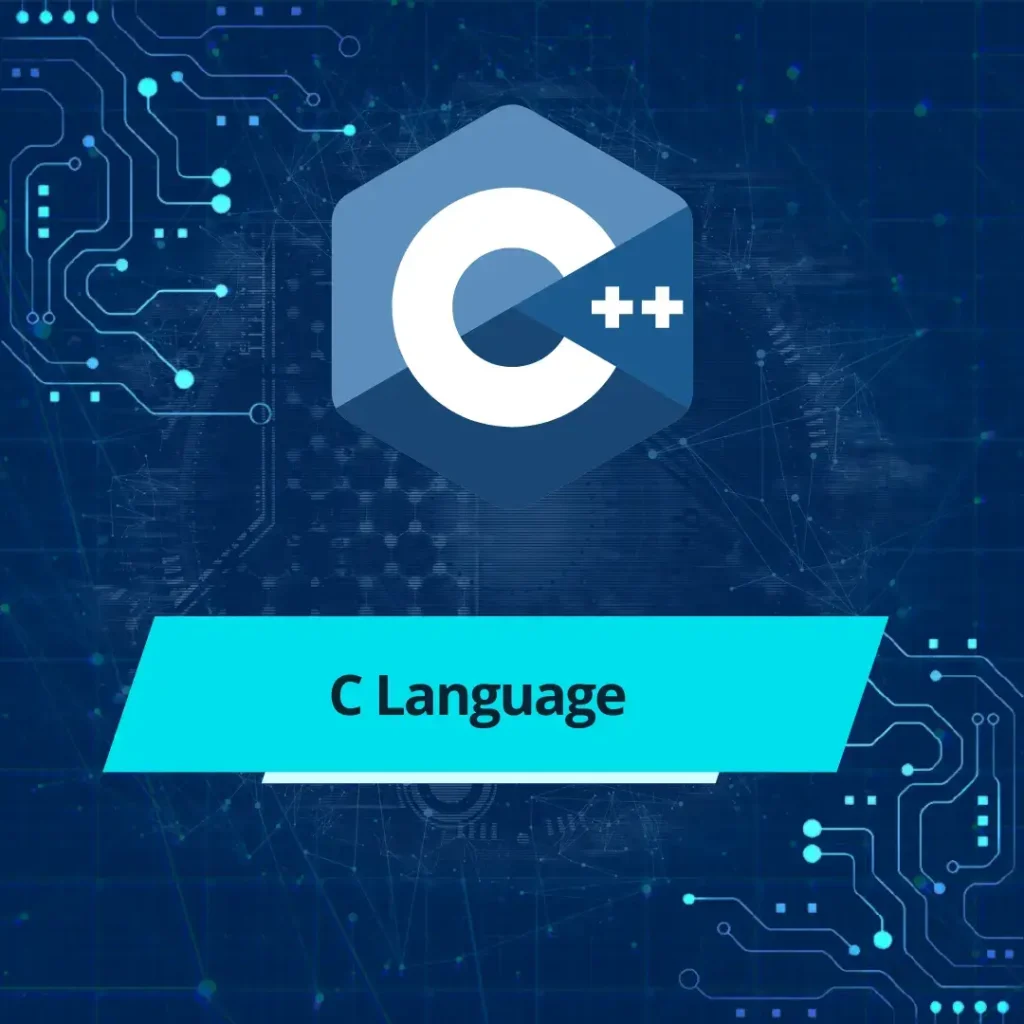
- Overview of the C++ programming language
- History and evolution of C++
- Setting up the development environment (GCC, Visual Studio, CLion)
- Writing and running your first C++ program
- Syntax and structure
- Data types: int, float, char, double, bool
- Variables and constants
- Basic input/output operations (cin, cout)
- Comments and documentation
- Arithmetic operators
- Relational and logical operators
- Bitwise operators
- Assignment operators
- Conditional (ternary) operator
- Operator precedence and associativity
- Conditional statements (if, if-else, nested if)
- Switch-case statement
- Looping constructs (for, while, do-while)
- Break and continue statements
- Function declaration and definition
- Calling functions
- Function parameters and return values
- Inline functions
- Function overloading
- Default arguments
- Local and global variables
- Static, extern, and register storage classes
- Scope and lifetime of variables
- Defining classes and creating objects
- Access specifiers (private, public, protected)
- Member functions and data members
- Constructors and destructors
this
pointer
- Base and derived classes
- Types of inheritance (single, multiple, multilevel, hierarchical)
- Overriding member functions
- Using
super
andthis
pointers - Virtual functions and polymorphism
- Abstract classes and pure virtual functions
- Interfaces and multiple inheritance
- Encapsulation and data hiding
- Operator overloading
- Friend functions and classes
- Function templates
- Class templates
- Template specialization
- STL (Standard Template Library) basics
- Try, catch, throw blocks
- Standard exceptions
- Custom exceptions
- Defining and using namespaces
- Nested namespaces
- The
using
directive
- Declaring and initializing arrays
- Multi-dimensional arrays
- String manipulation and functions
- Pointer basics and pointer arithmetic
- Dynamic memory allocation (new, delete)
- References and reference variables
- Smart pointers (unique_ptr, shared_ptr, weak_ptr)
- Opening and closing files
- Reading from and writing to files
- File handling functions (ifstream, ofstream, fstream)
- Binary file operations
- Random access to files
- Error handling in file operations
- Designing and implementing a C++ program
- Integrating various C++ programming concepts
- Testing and debugging the program
- Code review and presentation