Java
- Home
- Course
- Java
Introduction to Java
Java is a versatile and powerful programming language widely used in various domains, from web development to mobile applications. Our Java course module is designed to provide a thorough understanding of Java, catering to both beginners and experienced programmers looking to enhance their skills.
Duration - 2 Months
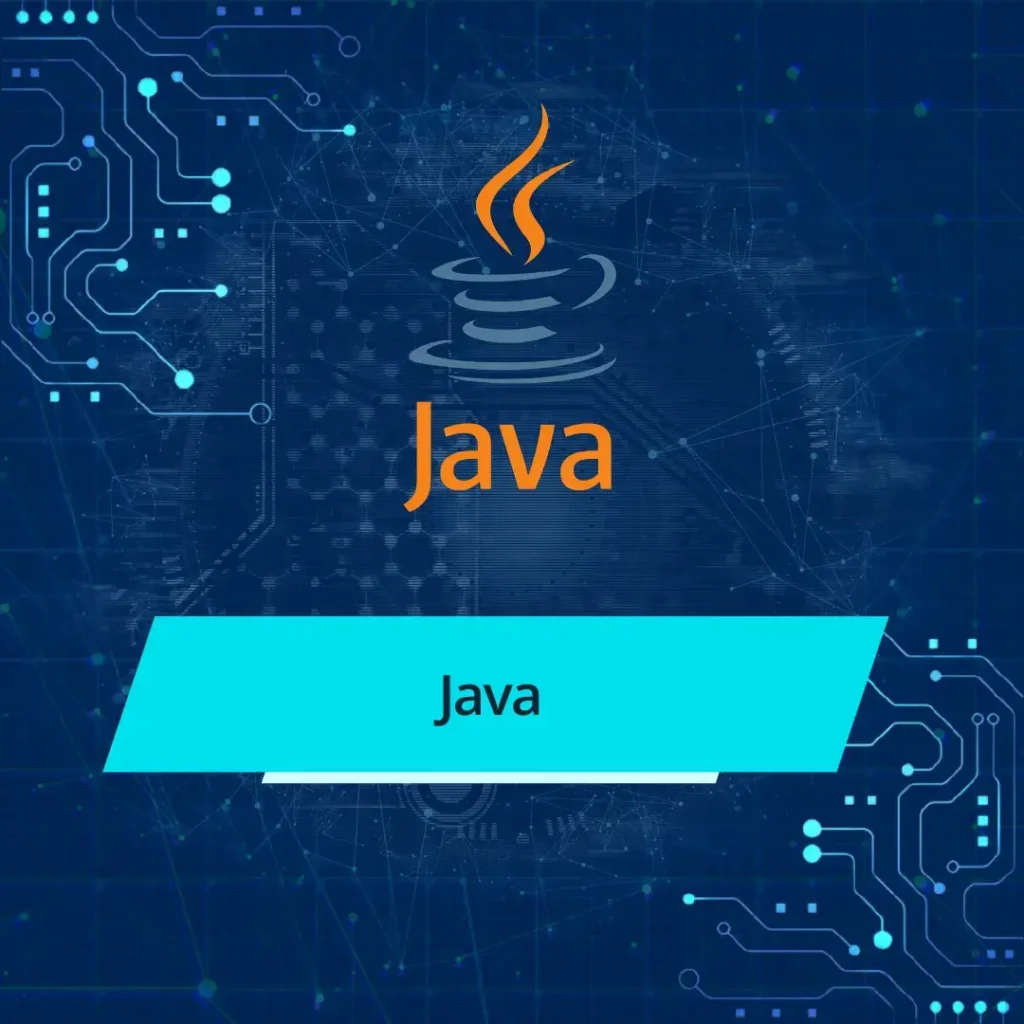
- Overview of Java programming language
- History and evolution of Java
- Java Development Kit (JDK) and Java Runtime Environment (JRE)
- Setting up the development environment (Eclipse, IntelliJ IDEA, or NetBeans)
- Java syntax and structure
- Data types, variables, and constants
- Operators and expressions
- Control flow statements (if-else, switch, loops)
- Principles of OOP (encapsulation, inheritance, polymorphism, abstraction)
- Classes and objects
- Constructors and methods
- Static keyword
- Inheritance and the super keyword
- Method overloading and overriding
- Abstract classes and interfaces
- Packages and access modifiers
- Overview of collections
- List, Set, and Map interfaces
- ArrayList, LinkedList, HashSet, TreeSet, HashMap, TreeMap
- Iterators and for-each loop
- Types of exceptions (checked and unchecked)
- Try-catch blocks and finally
- Throwing exceptions and custom exceptions
- Try-with-resources statement
- File class and its methods
- Reading and writing files using FileReader, FileWriter, BufferedReader, BufferedWriter
- Serialization and deserialization
- Designing and implementing a Java application
- Integrating various Java technologies
- Testing and debugging
- Deployment and presentation