Java Developer
- Home
- Courses
- Java Developer
Introduction to Java Developer
Understanding the fundamentals of Java development is crucial for anyone aspiring to become a proficient Java developer. This course module aims to equip learners with the foundational knowledge and skills necessary to excel in Java programming.
Duration - 4 Months
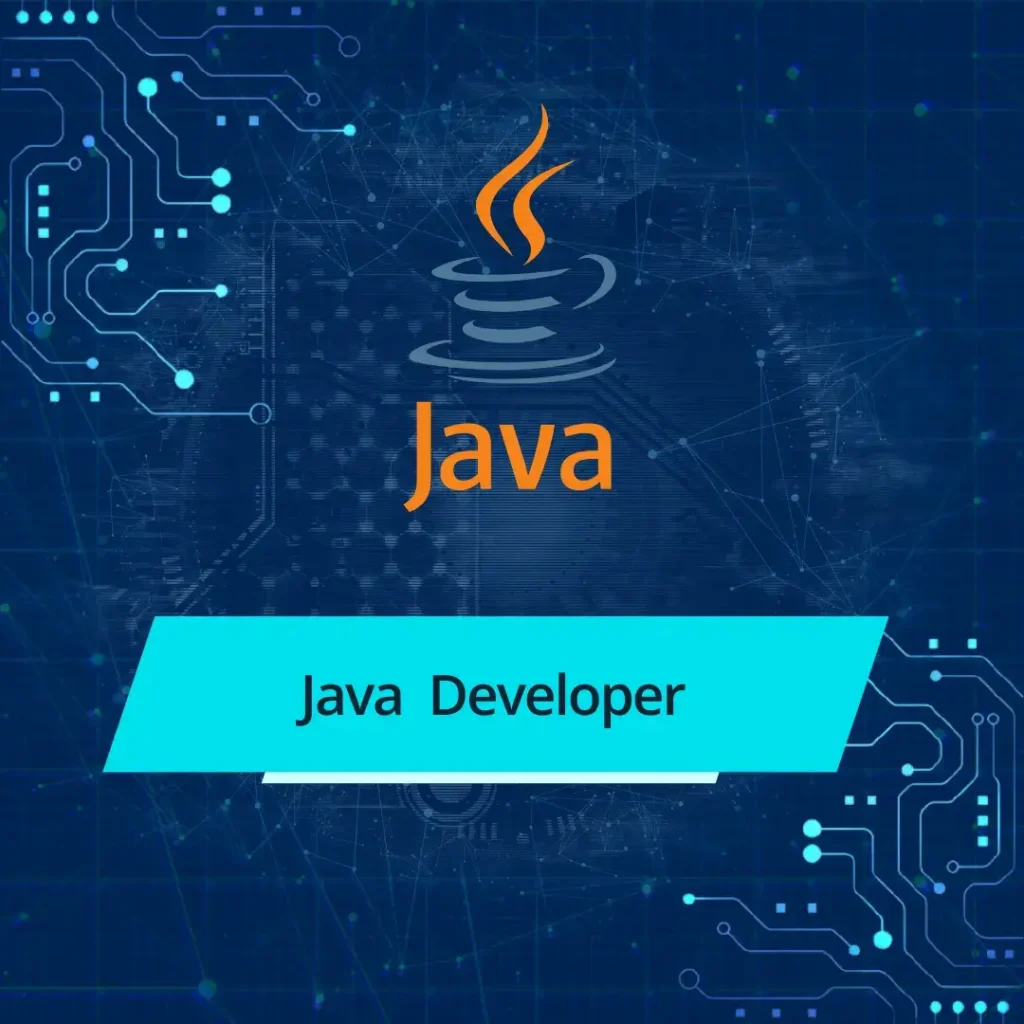
- Overview of Java programming language
- History and evolution of Java
- Java Development Kit (JDK) and Java Runtime Environment (JRE)
- Setting up the development environment (Eclipse, IntelliJ IDEA, or NetBeans)
- Java syntax and structure
- Data types, variables, and constants
- Operators and expressions
- Control flow statements (if-else, switch, loops)
- Principles of OOP (encapsulation, inheritance, polymorphism, abstraction)
- Classes and objects
- Constructors and methods
- Static keyword
- Inheritance and the super keyword
- Method overloading and overriding
- Abstract classes and interfaces
- Packages and access modifiers
- Overview of collections
- List, Set, and Map interfaces
- ArrayList, LinkedList, HashSet, TreeSet, HashMap, TreeMap
- Iterators and for-each loop
- Types of exceptions (checked and unchecked)
- Try-catch blocks and finally
- Throwing exceptions and custom exceptions
- Try-with-resources statement
- File class and its methods
- Reading and writing files using FileReader, FileWriter, BufferedReader, BufferedWriter
- Serialization and deserialization
- Introduction to generics
- Generic classes and methods
- Bounded types and wildcards
- Introduction to lambda expressions
- Functional interfaces
- Stream operations (filter, map, reduce)
- Collectors and parallel streams
- Introduction to threads and the Runnable interface
- Creating and managing threads
- Synchronization and thread safety
- Executors framework and Callable interface
- Introduction to Swing
- Creating windows and dialogs
- Event handling
- Layout managers
- Introduction to JavaFX
- Creating user interfaces with JavaFX
- Event handling and binding
- JavaFX controls and layouts
- Introduction to JDBC
- Connecting to a database
- CRUD operations with JDBC
- PreparedStatement and ResultSet
- Introduction to Hibernate
- Configuring Hibernate
- Mapping entities to database tables
- CRUD operations with Hibernate
- Introduction to web applications
- Writing servlets and handling requests
- JavaServer Pages (JSP) and JSTL
- Session management and cookies
- Designing and implementing a Java application
- Integrating various Java technologies
- Testing and debugging
- Deployment and presentation