Python
- Home
- Courses
- Python
Introduction to Python
Python is a versatile and high-level programming language that is extensively used for various applications, including web development, data analysis, artificial intelligence, and more. This Python course module is designed to provide a comprehensive understanding of Python, from the basics to advanced concepts.
Duration - 2 Months
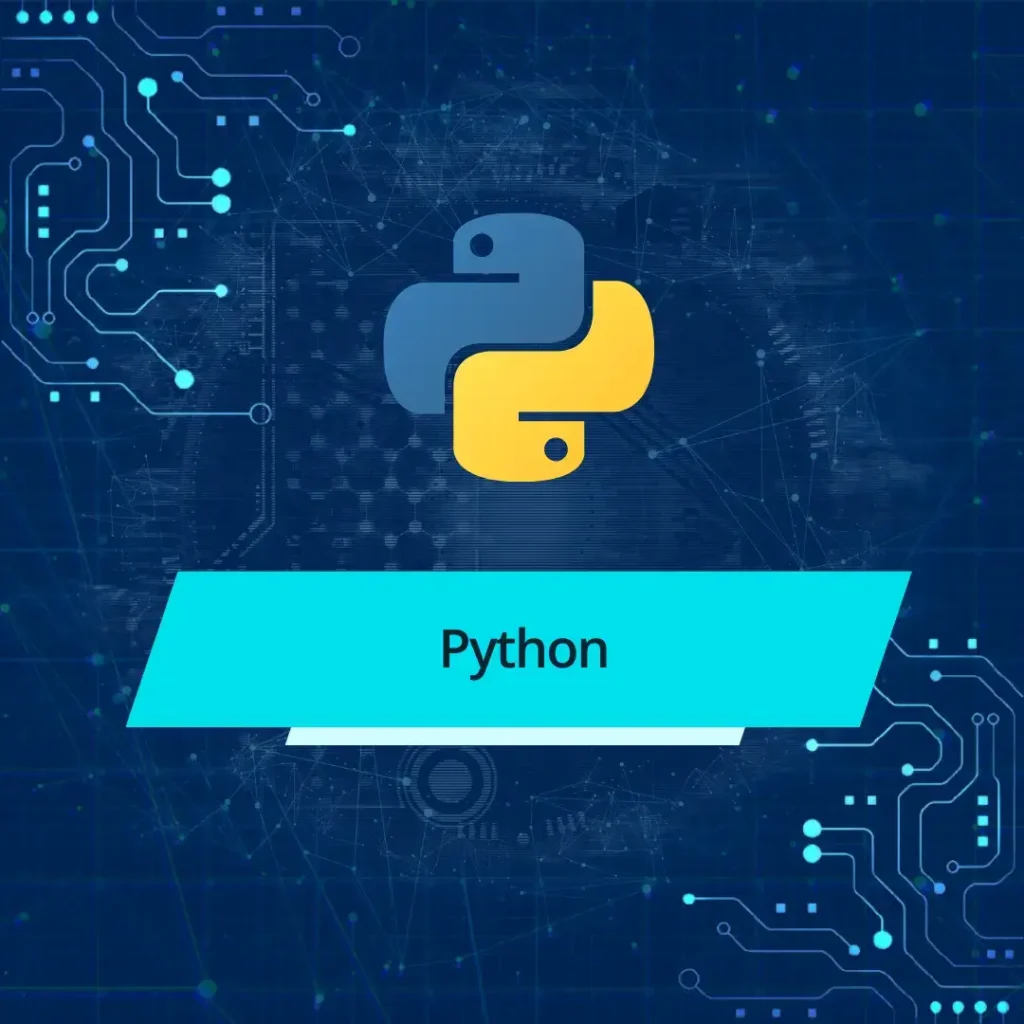
- Overview of Python programming language
- History and evolution of Python
- Installing Python and setting up the development environment (IDLE, PyCharm, VSCode)
- Writing and running your first Python scrip
- Python syntax and structure
- Data types: integers, floats, strings, booleans
- Variables and constants
- Basic input/output operations
- Comments and documentation
- Conditional statements (if, elif, else)
- Looping constructs (for, while)
- Break, continue, and pass statements
- Defining and calling functions
- Function parameters and return values
- Lambda functions
- Variable scope and namespaces
- Creating and accessing list elements
- List operations and methods
- List comprehensions
- Creating and accessing tuple elements
- Tuple operations
- Using tuples for multiple assignments
- Creating and accessing dictionary elements
- Dictionary operations and methods
- Iterating over dictionaries
- Creating and accessing set elements
- Set operations and methods
- Working with sets
- Defining classes and creating objects
- Instance variables and methods
- The
self
parameter
- Inheriting from a base class
- Overriding methods
- Using
super()
- Method overloading and overriding
- Encapsulation and data hiding
- Properties and property decorators
- Importing modules
- Creating and using custom modules
- Standard library modules (math, datetime, os, sys)
- Creating and using packages
- Understanding
__init__.py
- Managing package imports
- Opening and closing files
- Reading from and writing to files
- Working with file paths
- Understanding exceptions
- Using try, except, else, finally blocks
- Raising exceptions
- Creating custom exceptions
- Designing and implementing a Python application
- Integrating various Python technologies
- Testing and debugging the application
- Deploying the application