Spring Boot
- Home
- Courses
- Spring boot
Introduction to Spring Boot
The Spring Boot framework is designed to simplify the development of production-ready applications by providing a suite of tools and default configurations. This course module will introduce you to the essentials of Spring Boot, including its architecture, core features, and how it integrates with other Spring projects.
Duration - 6 Months
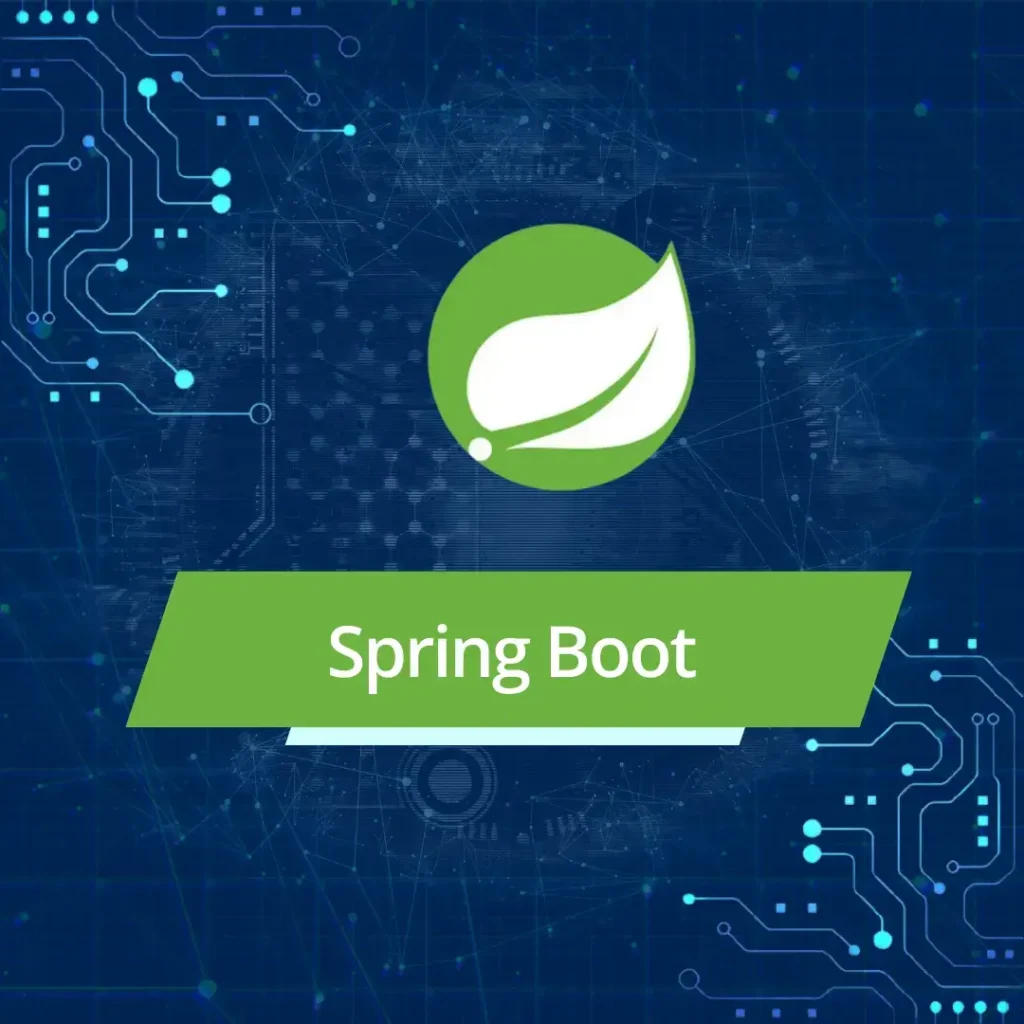
- Overview of Spring Framework
- Introduction to Spring Boot
- Advantages of using Spring Boot
- Setting up the development environment
- Creating a Spring Boot project
- Spring Boot project structure
- Configuration with Spring Boot
- Running a Spring Boot application
- Dependency Injection and Inversion of Control
- Spring Boot Starters
- Spring Boot Auto-configuration
- Application Properties and YAML Configuration
- Introduction to REST
- Creating RESTful endpoints
- Handling HTTP methods (GET, POST, PUT, DELETE)
- Path variables and request parameters
- Error handling and exception management
- Introduction to Spring Data JPA
- Configuring a data source
- CRUD operations with JPA repositories
- Using Spring Boot with Hibernate
- Pagination and Sorting
- Connecting to relational databases (MySQL, PostgreSQL)
- Database migrations with Flyway and Liquibase
- Working with NoSQL databases (MongoDB)
- Using Spring Data MongoDB
- Introduction to Spring Security
- Configuring authentication and authorization
- Securing REST APIs
- JWT (JSON Web Token) authentication
- Role-based access control
- Unit testing with JUnit and Mockito
- Integration testing with Spring Boot
- Testing RESTful services
- Testcontainers for database integration testing
- Designing and implementing a Spring Boot application
- Integrating multiple modules and components
- End-to-end testing and validation
- Deployment and presentation